Software Engineering
I am very interested in going to college for software engineering. I have created software before in VB.NET, and have always had an interest in software and computers. My question is, what things are taught in a software engineering class, and what languages and other things should I learn/do to be prepared for the class? #computer #software #software-engineer
4 answers
Robert’s Answer
What gets taught will vary widely based on what type of school you attend and what course work you take. But some things you should expect include:
Freshman / Sophomore Year
- Math, Science and Logic - a BS program will probably include Calc I, II and discrete math. Possibly stats. A BA program will probably only be Calc 1.
- Basic Computing - schools can't assume you know anything about computers so this is laying down a foundation
- Basic Programming - probably in a language like Java or Python.
- Basic Algorithms and Data Structures - the big ones. Lists, stacks, queues, trees (balanced and not), basic graphs, sorting and searching.
Junior / Senior Year
- More math and science - probably through Calc III. Stats if you didn't get it earlier.
- Programming Electives. Operating systems, compilers, graphics, data, analytics, etc. This is where you will have more freedom to pick your own topics
- Senior projects - many schools require these
- Internships - this is the whole point of the previous 2 or 3 years. Seriously. It is.
Pick a few schools and look at their course work by semester. Here's NCSU's breakdown for computer science:
https://www.csc.ncsu.edu/academics/undergrad/semester.php
This should give you an idea of what is coming.
If you really want to get ahead of the curve I would not worry about things like learning more programming languages or website design or the hot new thing. Start with the fundamentals. Those transcend language and hotnewcoolness. They teach you how to think. They lay a foundation.
Try this in the language of your choice. If you want me to suggest one - I suggest Java or C#. You don't need to know very much of the base language to do these things.
Data Structure Basics:
- Create a linked list class
- Implement a Stack and Queue using the linked list as the underlying storage
- Create a binary search tree
- Create a set class using your binary search tree. Implement union, intersection, difference and symmetric difference algorithms
Algorithm Basics
- Implement bubble, selection, insertion, merge and quick sort. Really take the time to understand how they work and in what case you might want one or the other.
- Implement binary search over an array/vector.
- Implement the Boyer Moore string matching algorithm
For each of those 7 points make sure you understand the "Big-O" order of everything. Understanding operational complexity is the thing that matters.
If you went into your freshman year knowing these things then you will know more than what many people remember 10 years after college.
Kirby’s Answer
Robert gave a great overview of what pretty much any Software Engineering program will be like. However, if you really want to prepare for being a software engineer (instead of preparing for the classes) I'd recommend focusing on the really important concepts they don't spend a lot of time on in school.
I would recommend spending time getting familiar with using version control, continuous integration tools, writing unit and integration tests, learning about code coverage, and treating your school projects like a system that needs to be designed in an environment where you're not the only one working on the code. This means clarifying requirements, noting assumptions, writing clean code, commenting your code, planning on paper/whiteboard/chalkboard before you even touch your keyboard.
You won't really need to use any of these concepts in your undergrad degree but they are all crucial to working as a professional developer. Don't worry if this feels overwhelming. You don't need to worry about learning these concepts until after you feel comfortable with what you're doing in classes. But if you ever find yourself thinking that what you're learning is too easy or if it feels like you already know it, spend some time learning about/implementing these concepts. It will pay off in the long run.
Good luck! You've got a fun journey ahead of you!
Michael’s Answer
I would highlight what Kirby said, but focus on version control, such as git, because you can begin using it fairly easily, it makes group coding for a class easier, and every single position in software requires you to know it.
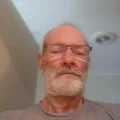
James Constantine Frangos
James Constantine’s Answer
Software engineering courses offer a comprehensive overview of the principles, methodologies, and technologies vital to software development. Here's a breakdown of key subjects you'll likely encounter:
1. Programming Languages: You'll be introduced to popular languages like Java, C++, Python, etc. Grasping their syntax, semantics, and best practices is fundamental for software engineers.
2. Software Development Methodologies: You'll explore methodologies such as Agile, Waterfall, Scrum, and DevOps, learning how to plan, design, execute, test, and maintain software projects.
3. Data Structures and Algorithms: Courses often emphasize essential data structures (like arrays, linked lists, trees) and algorithms (like sorting and searching) for creating efficient, scalable code.
4. Database Management: You'll delve into database concepts, SQL queries, database design, normalization techniques, and interacting with databases in software projects.
5. Software Design Patterns: Familiarizing yourself with common patterns like MVC (Model-View-Controller), Singleton, Factory Method, etc., aids in writing modular, maintainable code.
6. Software Testing: Quality assurance and testing are integral, teaching you various techniques, tools, and strategies to ensure software reliability and functionality.
7. Operating Systems: You'll learn the basics of operating systems to understand how software and hardware interact.
8. Project Management: Some courses cover project management, teaching you how to effectively manage software projects within time and budget limits.
To excel in a software engineering course, it's advantageous to have a good grasp of at least one programming language (like VB.NET), basic knowledge of data structures and algorithms, an understanding of software development concepts, and strong problem-solving skills.
Three authoritative sources for further study include:
1. IEEE Computer Society: A leading resource offering insights into computer science and software engineering education.
2. Association for Computing Machinery (ACM): A top organization providing valuable information on computer science education, including software engineering curriculum guidelines and best practices.
3. Coursera - Software Engineering Courses: Offers online courses from top global universities on software engineering topics, giving insights into what traditional software engineering classes cover.
By exploring these resources, you'll gain a deeper understanding of what's typically taught in software engineering classes and be better equipped for your academic journey in this field.