4 answers
4 answers
Updated
Daria’s Answer
Here are some tips to help you code effectively:
1. Make your fundamentals clear: Skipping the basics and jumping to advanced concepts can lead to confusion and frustration. Start with one programming language, stick with it, and clear all the basics of programming first before moving to the next level.
2. Learn by doing: Reading about loops and variables is not enough. You need to get your hands dirty in coding and keep practicing it regularly. When you write code, play with it, change it to see different results, optimize it, and try different solutions. Your logical thinking ability will improve day by day, and you will eventually learn a lot of things that make you a better programmer.
3. Use code editors in your smartphone: Having a PC or laptop every time along with you is not always possible. But due to this, your learning progress should not be affected or slowed down. Use some good code editors in your smartphones to continue your learning and practice sessions.
4. Use a coding standard: Writing bad, unorganized code is easy, but maintaining such code is hard. Use a coding standard to write clean, organized, and maintainable code.
5. Write useful comments: Comments are crucial. They help you and others understand the code better. Write comments that explain what the code does, why it does it, and how it does it.
6. Refactor code: Refactoring is the eighth habit of highly effective developers. It means improving the code's design without changing its functionality. Refactoring makes the code more readable, maintainable, and efficient.
7. Avoid global code: Global code is code that is not inside any function or class. It can cause naming conflicts, security issues, and make the code harder to maintain. Avoid using global code as much as possible.
8. Use meaningful names: Use meaningful names for variables, functions, classes, and other entities in your code. Meaningful names make the code more readable and understandable.
9. Use version control software: Version control software helps you keep track of changes to your code, collaborate with others, and revert to previous versions if needed. Git is a popular version control software that you can use.
10. Use automated build tools: Automated build tools help you automate the process of building, testing, and deploying your code. They save you time and effort and reduce the chances of human error. Some popular automated build tools are Jenkins, Travis CI, and CircleCI.
Remember, learning to code and mastering it can take years for a beginner. It requires dedication, passion, interest, and patience. But with the right mindset and approach, you can learn to code easily, quickly, and effectively.
Additionally, besides working on your code skills, focus on doing good research on IDE you are using. The more you know tricks in your environment the easier and more efficient your coding will be.
1. Make your fundamentals clear: Skipping the basics and jumping to advanced concepts can lead to confusion and frustration. Start with one programming language, stick with it, and clear all the basics of programming first before moving to the next level.
2. Learn by doing: Reading about loops and variables is not enough. You need to get your hands dirty in coding and keep practicing it regularly. When you write code, play with it, change it to see different results, optimize it, and try different solutions. Your logical thinking ability will improve day by day, and you will eventually learn a lot of things that make you a better programmer.
3. Use code editors in your smartphone: Having a PC or laptop every time along with you is not always possible. But due to this, your learning progress should not be affected or slowed down. Use some good code editors in your smartphones to continue your learning and practice sessions.
4. Use a coding standard: Writing bad, unorganized code is easy, but maintaining such code is hard. Use a coding standard to write clean, organized, and maintainable code.
5. Write useful comments: Comments are crucial. They help you and others understand the code better. Write comments that explain what the code does, why it does it, and how it does it.
6. Refactor code: Refactoring is the eighth habit of highly effective developers. It means improving the code's design without changing its functionality. Refactoring makes the code more readable, maintainable, and efficient.
7. Avoid global code: Global code is code that is not inside any function or class. It can cause naming conflicts, security issues, and make the code harder to maintain. Avoid using global code as much as possible.
8. Use meaningful names: Use meaningful names for variables, functions, classes, and other entities in your code. Meaningful names make the code more readable and understandable.
9. Use version control software: Version control software helps you keep track of changes to your code, collaborate with others, and revert to previous versions if needed. Git is a popular version control software that you can use.
10. Use automated build tools: Automated build tools help you automate the process of building, testing, and deploying your code. They save you time and effort and reduce the chances of human error. Some popular automated build tools are Jenkins, Travis CI, and CircleCI.
Remember, learning to code and mastering it can take years for a beginner. It requires dedication, passion, interest, and patience. But with the right mindset and approach, you can learn to code easily, quickly, and effectively.
Additionally, besides working on your code skills, focus on doing good research on IDE you are using. The more you know tricks in your environment the easier and more efficient your coding will be.
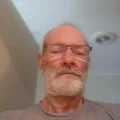
James Constantine Frangos
Consultant Dietitian & Software Developer since 1972 => Nutrition Education => Health & Longevity => Self-Actualization.
5265
Answers
Updated
James Constantine’s Answer
Hey there, Kate!
Let's dive right into the world of Coding!
Think of coding, or programming, as a recipe for your computer. It's all about crafting a set of instructions that your computer can follow. These instructions, written in various programming languages, help create software, websites, and apps. To be a whiz at coding, there are a few key concepts and skills you need to get your head around.
1. Programming Languages: Just like there are different languages around the world, there are different programming languages too! Each one has its own grammar, rules, and ideal uses. Some of the big names you might have heard of are Python, Java, JavaScript, C++, and Ruby. Picking the right language depends on what you want to achieve and the projects you're keen to work on.
2. Algorithms and Data Structures: These are the building blocks of efficient and optimized code. Algorithms are like a roadmap for solving problems, while data structures are the containers that hold and organize data. Mastering these can really boost the performance and scalability of your code.
3. Problem-Solving Skills: Coding is all about solving puzzles. You need to be able to break down big, complex problems into smaller, more manageable chunks and come up with logical solutions. This means understanding what's needed, spotting potential roadblocks, and figuring out the best way to tackle them.
4. Version Control Systems: Tools like Git are a coder's best friend. They help manage changes to your code, make collaboration easier, and keep a record of all your modifications. Knowing how to use version control is key when working in a team and keeping your codebase in good shape.
5. Integrated Development Environments (IDEs): These are like your coding toolbox. IDEs are software applications packed with features like code editors, debuggers, and build automation tools. They make the coding process smoother and can boost your productivity by providing a one-stop-shop for all your development needs.
How to Learn Coding:
There's a wealth of resources and tools out there to help you become a coding pro:
1. Online Learning Platforms: Websites like Codecademy, Coursera, Udemy, and Khan Academy offer interactive courses on a range of programming languages and computer science basics. They provide structured learning paths, hands-on exercises, and assessments to help you get to grips with coding.
2. Documentation and Tutorials: Official guides for programming languages and frameworks are a goldmine of information. Plus, there are heaps of tutorials on sites like W3Schools, MDN Web Docs (Mozilla Developer Network), and Stack Overflow that offer practical advice on coding techniques and best practices.
3. Community Support: Joining developer communities on platforms like Stack Overflow, GitHub, and Reddit can be a great way to learn from seasoned programmers, get advice on coding challenges, and keep up-to-date with the latest trends.
4. Code Editors: Tools like Visual Studio Code, Sublime Text, or Atom can make coding a breeze. They offer features like syntax highlighting, auto-completion, and debugging capabilities.
5. Project-Based Learning: The best way to learn is by doing. Building real-world projects lets you put your coding skills to the test. Sites like GitHub have repositories full of open-source projects where you can contribute and collaborate with other developers.
By getting a handle on these key concepts and making the most of the available learning tools, you'll be well on your way to building a solid foundation in coding. This will equip you to take on a wide range of programming challenges with confidence.
Top 3 References Used:
Codecademy: A popular online platform offering interactive coding courses in various programming languages.
MDN Web Docs (Mozilla Developer Network): A comprehensive resource for documentation and tutorials related to web development technologies.
GitHub: A leading platform for version control using Git and a hub for collaborative software development projects.
These sources were used to gather info on effective coding practices, learning resources, and industry-standard tools.
Take care and happy coding!
Best,
James.
Let's dive right into the world of Coding!
Think of coding, or programming, as a recipe for your computer. It's all about crafting a set of instructions that your computer can follow. These instructions, written in various programming languages, help create software, websites, and apps. To be a whiz at coding, there are a few key concepts and skills you need to get your head around.
1. Programming Languages: Just like there are different languages around the world, there are different programming languages too! Each one has its own grammar, rules, and ideal uses. Some of the big names you might have heard of are Python, Java, JavaScript, C++, and Ruby. Picking the right language depends on what you want to achieve and the projects you're keen to work on.
2. Algorithms and Data Structures: These are the building blocks of efficient and optimized code. Algorithms are like a roadmap for solving problems, while data structures are the containers that hold and organize data. Mastering these can really boost the performance and scalability of your code.
3. Problem-Solving Skills: Coding is all about solving puzzles. You need to be able to break down big, complex problems into smaller, more manageable chunks and come up with logical solutions. This means understanding what's needed, spotting potential roadblocks, and figuring out the best way to tackle them.
4. Version Control Systems: Tools like Git are a coder's best friend. They help manage changes to your code, make collaboration easier, and keep a record of all your modifications. Knowing how to use version control is key when working in a team and keeping your codebase in good shape.
5. Integrated Development Environments (IDEs): These are like your coding toolbox. IDEs are software applications packed with features like code editors, debuggers, and build automation tools. They make the coding process smoother and can boost your productivity by providing a one-stop-shop for all your development needs.
How to Learn Coding:
There's a wealth of resources and tools out there to help you become a coding pro:
1. Online Learning Platforms: Websites like Codecademy, Coursera, Udemy, and Khan Academy offer interactive courses on a range of programming languages and computer science basics. They provide structured learning paths, hands-on exercises, and assessments to help you get to grips with coding.
2. Documentation and Tutorials: Official guides for programming languages and frameworks are a goldmine of information. Plus, there are heaps of tutorials on sites like W3Schools, MDN Web Docs (Mozilla Developer Network), and Stack Overflow that offer practical advice on coding techniques and best practices.
3. Community Support: Joining developer communities on platforms like Stack Overflow, GitHub, and Reddit can be a great way to learn from seasoned programmers, get advice on coding challenges, and keep up-to-date with the latest trends.
4. Code Editors: Tools like Visual Studio Code, Sublime Text, or Atom can make coding a breeze. They offer features like syntax highlighting, auto-completion, and debugging capabilities.
5. Project-Based Learning: The best way to learn is by doing. Building real-world projects lets you put your coding skills to the test. Sites like GitHub have repositories full of open-source projects where you can contribute and collaborate with other developers.
By getting a handle on these key concepts and making the most of the available learning tools, you'll be well on your way to building a solid foundation in coding. This will equip you to take on a wide range of programming challenges with confidence.
Top 3 References Used:
Codecademy: A popular online platform offering interactive coding courses in various programming languages.
MDN Web Docs (Mozilla Developer Network): A comprehensive resource for documentation and tutorials related to web development technologies.
GitHub: A leading platform for version control using Git and a hub for collaborative software development projects.
These sources were used to gather info on effective coding practices, learning resources, and industry-standard tools.
Take care and happy coding!
Best,
James.
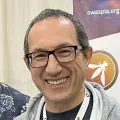
Edmond Momartin ☁️
Public Cloud Security & Compliance AT&T | MBA InfoSec | OWASP-LA Board
68
Answers
Updated
Edmond’s Answer
One of the best sources to help you get started coding are https://code.org/ and https://www.khanacademy.org/hourofcode
If you already know how to code and want to improve your skill, I'd suggest YouTube, Codecademy, freeCodeCamp, etc.
-edmond
If you already know how to code and want to improve your skill, I'd suggest YouTube, Codecademy, freeCodeCamp, etc.
-edmond
Updated
Bill’s Answer
This is sort of an experimental approach but I've found it great for me. I learn by doing. I find that having something I want to do and figuring out how moves me forward much more quickly than sitting through a class.
I would suggest that you get on chatGPT (chat.openai.com) and start with something very simple. Once you've done this you can try it in different languages like Javascript and add extra things to try. Has a chatGPT transcript for a very simple example to get started. Then I've added something with a little more going on to sort some names. Study the output to understand what the program is doing. You can ask chatGPT to explain why each line is there.
EXAMPLE #1
Me
Please code a "Hello world" program for me in Python and show me how to run it on my computer.
ChatGPT
I have written and saved a simple "Hello, World!" program in Python for you. Here's the code:
python
Copy code
# This is a simple Python program that prints "Hello, World!" to the console
print("Hello, World!")
You can download the Python file from the following link: hello_world.py.
To run this program on your computer, follow these steps:
Download and Install Python (if you haven't already):
Visit the official Python website: python.org
Download the latest version of Python for your operating system (Windows, macOS, Linux).
Install Python by following the installation wizard.
Save the Python File:
Download the hello_world.py file from the link above.
Save it to a convenient location on your computer.
Run the Program:
Open your computer's terminal or command prompt.
Navigate to the directory where you saved the hello_world.py file. You can use the cd command followed by the directory path to do this.
Type python hello_world.py and press Enter to run the program.
You should see Hello, World! printed in the terminal.
Let me know if you need any more help with this!
EXAMPLE #2
Me
Please write me a program in Python to read in names that I type in, sort them, and print out the list. Show me how to run Python on my Windows computer.
ChatGPT
Certainly! I'll guide you through the process of writing a simple Python program to read in names, sort them, and print out the sorted list. Then, I'll explain how to run Python on your Windows computer.
Python Program
Create a Python File:
First, open a text editor (like Notepad) and paste the following Python code into it.
Save the file with a .py extension, for example, sort_names.py.
python
Copy code
def main():
print("Enter names (type 'done' when finished):")
names = []
while True:
name = input()
if name.lower() == 'done':
break
names.append(name)
names.sort()
print("\nSorted Names:")
for name in names:
print(name)
if __name__ == "__main__":
main()
This program will prompt you to enter names one by one. When you're finished, type done, and it will display the sorted list of names.
Running the Program on Windows
Install Python (if not already installed):
Go to the official Python website.
Download the latest version for Windows.
Run the installer. Make sure to check the box that says "Add Python to PATH" during installation.
Run the Program:
Open Command Prompt.
Navigate to the directory where your sort_names.py file is saved. Use the cd command to change directories. For example, if your file is in Documents, type cd Documents.
Run the program by typing python sort_names.py and pressing Enter.
You will then be able to enter names as prompted by the program. Once you type done, it will display the sorted list of names.
I would suggest that you get on chatGPT (chat.openai.com) and start with something very simple. Once you've done this you can try it in different languages like Javascript and add extra things to try. Has a chatGPT transcript for a very simple example to get started. Then I've added something with a little more going on to sort some names. Study the output to understand what the program is doing. You can ask chatGPT to explain why each line is there.
EXAMPLE #1
Me
Please code a "Hello world" program for me in Python and show me how to run it on my computer.
ChatGPT
I have written and saved a simple "Hello, World!" program in Python for you. Here's the code:
python
Copy code
# This is a simple Python program that prints "Hello, World!" to the console
print("Hello, World!")
You can download the Python file from the following link: hello_world.py.
To run this program on your computer, follow these steps:
Download and Install Python (if you haven't already):
Visit the official Python website: python.org
Download the latest version of Python for your operating system (Windows, macOS, Linux).
Install Python by following the installation wizard.
Save the Python File:
Download the hello_world.py file from the link above.
Save it to a convenient location on your computer.
Run the Program:
Open your computer's terminal or command prompt.
Navigate to the directory where you saved the hello_world.py file. You can use the cd command followed by the directory path to do this.
Type python hello_world.py and press Enter to run the program.
You should see Hello, World! printed in the terminal.
Let me know if you need any more help with this!
EXAMPLE #2
Me
Please write me a program in Python to read in names that I type in, sort them, and print out the list. Show me how to run Python on my Windows computer.
ChatGPT
Certainly! I'll guide you through the process of writing a simple Python program to read in names, sort them, and print out the sorted list. Then, I'll explain how to run Python on your Windows computer.
Python Program
Create a Python File:
First, open a text editor (like Notepad) and paste the following Python code into it.
Save the file with a .py extension, for example, sort_names.py.
python
Copy code
def main():
print("Enter names (type 'done' when finished):")
names = []
while True:
name = input()
if name.lower() == 'done':
break
names.append(name)
names.sort()
print("\nSorted Names:")
for name in names:
print(name)
if __name__ == "__main__":
main()
This program will prompt you to enter names one by one. When you're finished, type done, and it will display the sorted list of names.
Running the Program on Windows
Install Python (if not already installed):
Go to the official Python website.
Download the latest version for Windows.
Run the installer. Make sure to check the box that says "Add Python to PATH" during installation.
Run the Program:
Open Command Prompt.
Navigate to the directory where your sort_names.py file is saved. Use the cd command to change directories. For example, if your file is in Documents, type cd Documents.
Run the program by typing python sort_names.py and pressing Enter.
You will then be able to enter names as prompted by the program. Once you type done, it will display the sorted list of names.